初めに
画像から犬種・猫種を特定したいと思います。それもたった11行のpythonのコードで!
tensorflowには、学習済みのモデルがたくさん組み込まれています。その中のefficient netを使用します。このモデルはすでに1000種類の画像分類タスクを学習されているので、自分で学習させる必要なくすぐに分類に使用できます。このモデルでは、犬は125種、猫は5種の分類ができます。
tensorflowで使用できるモデル一覧はこちら
https://www.tensorflow.org/api_docs/python/tf/keras/applications
モデルが多すぎてどれを使ったらいいのかわからないですが、以下のQiita記事がヒントになります。
https://qiita.com/omiita/items/83643f78baabfa210ab1
コード
google colabを使うと環境設定も不要で、簡単です。以下のようにcolabの画面の左下のところに写真をドロップしてください。
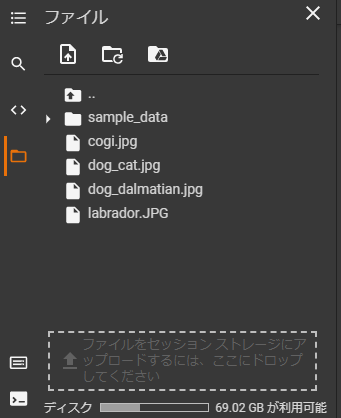
この写真を判定してもらいます。
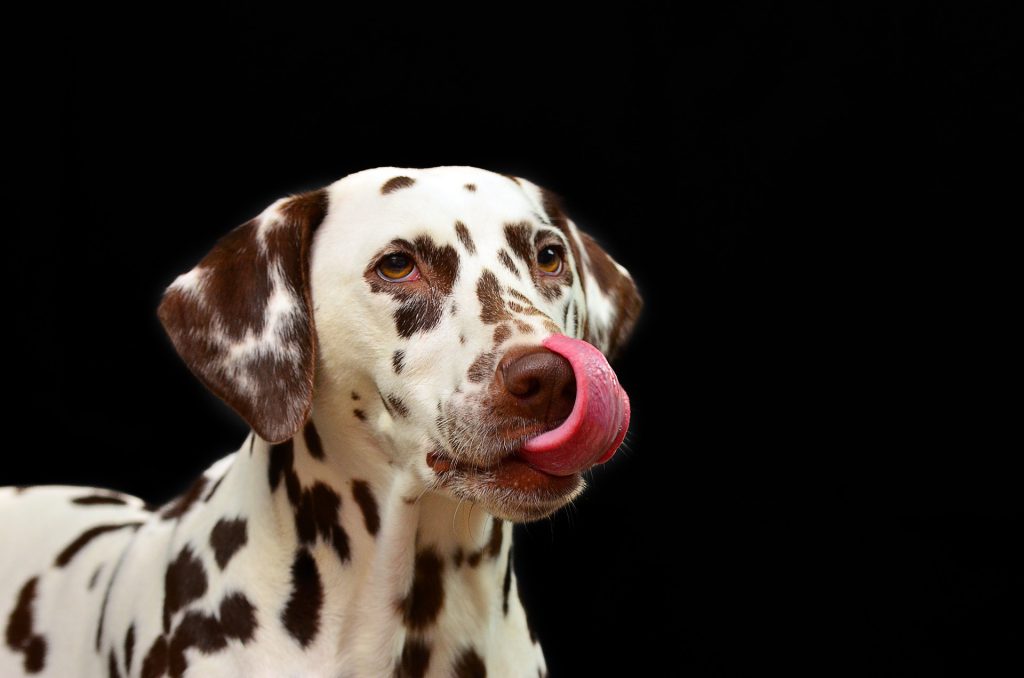
商用利用無料
Pixabay License
帰属表示は必要ありません
そして、以下のコードを実行します。
from tensorflow.keras.applications import EfficientNetB0
from tensorflow.keras.preprocessing.image import load_img, img_to_array
import numpy as np
import tensorflow as tf
# 写真の前処理を行う※
pic = load_img("/content/dog_dalmatian.jpg", target_size= (224,224,3))
pic = img_to_array(pic).astype('float32')
pic = np.expand_dims(pic,axis=0)
processedpic = tf.keras.applications.efficientnet.preprocess_input(pic)
# efficientnetのモデルを読み込む
model = EfficientNetB0(weights='imagenet')
# modelを使って犬種・猫種を予想する
prediction = model.predict(processedpic)
tf.keras.applications.efficientnet.decode_predictions(prediction)
[[(‘n02110341’, ‘dalmatian’, 0.90001744),
(‘n02109047’, ‘Great_Dane’, 0.0051623993),
(‘n02100735’, ‘English_setter’, 0.0027725038),
(‘n02100236’, ‘German_short-haired_pointer’, 0.0025760268),
(‘n02092339’, ‘Weimaraner’, 0.0019489381)]]
1000カテゴリーの中から可能性が高い5カテゴリーを確率で教えてくれます。1000カテゴリーは、犬以外のカテゴリもたくさん入っています。
ダルメシアンが90%です!正解!
load_img()の中には、写真のファイルのパスを設定してください。
他にも試してみます。
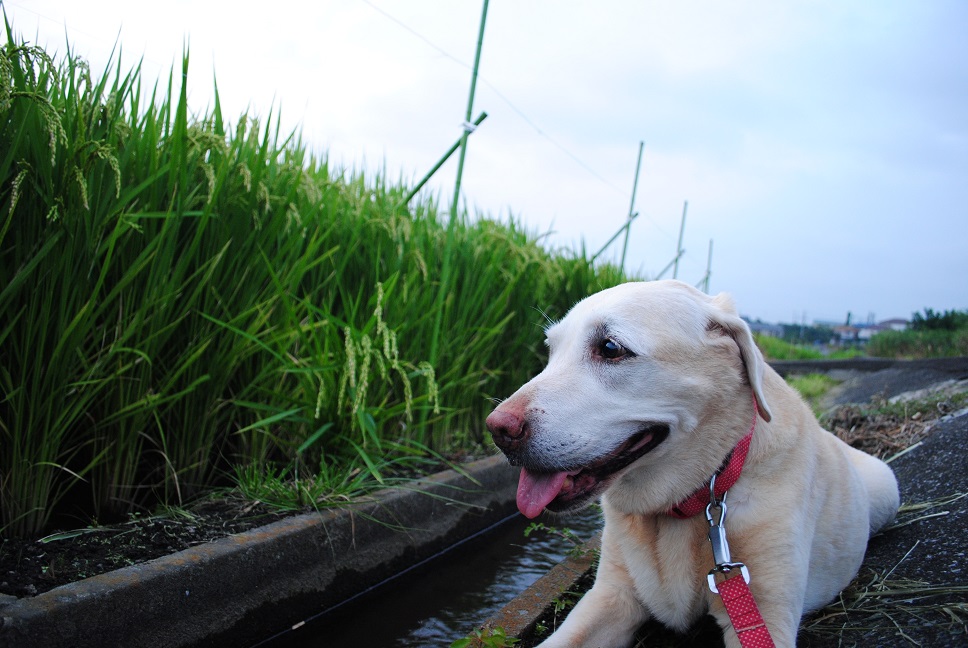
[[(‘n02099712’, ‘Labrador_retriever’, 0.42502728),
(‘n02093428’, ‘American_Staffordshire_terrier’, 0.04134651),
(‘n02109961’, ‘Eskimo_dog’, 0.032269508),
(‘n02110185’, ‘Siberian_husky’, 0.031741217),
(‘n02093256’, ‘Staffordshire_bullterrier’, 0.024241285)]]
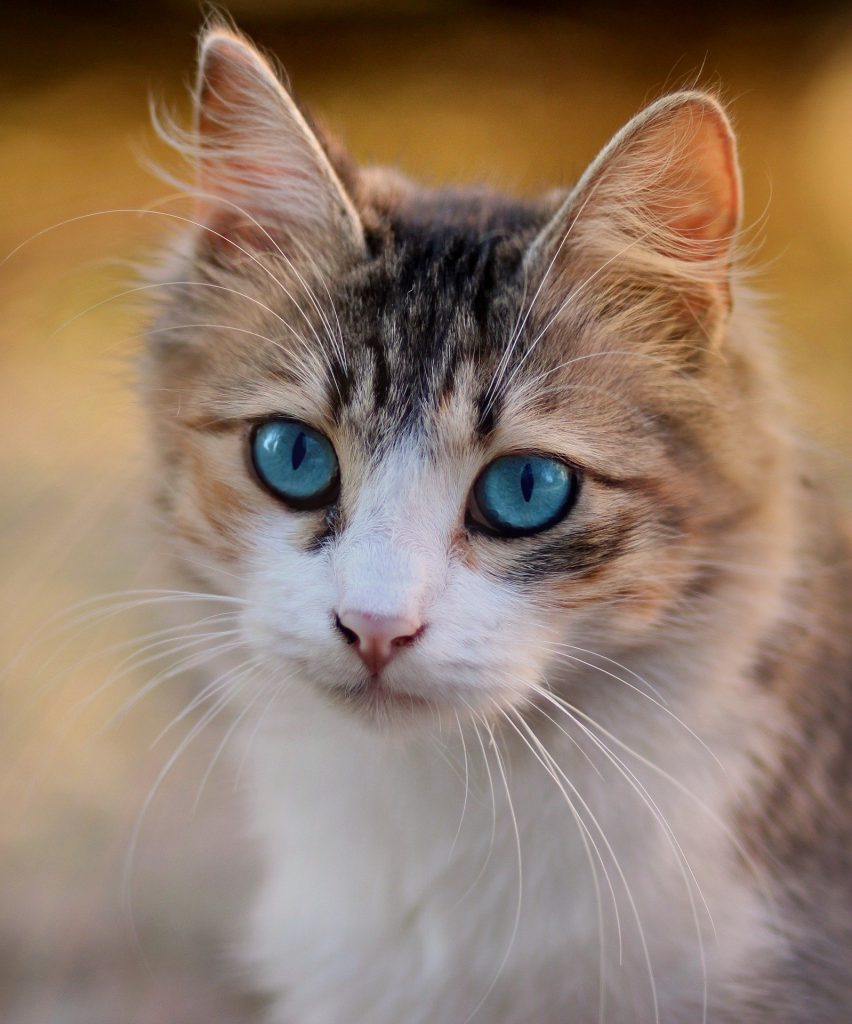
[[(‘n02123045’, ‘tabby’, 0.23962992),
(‘n02123159’, ‘tiger_cat’, 0.21859926),
(‘n02127052’, ‘lynx’, 0.13928987),
(‘n02124075’, ‘Egyptian_cat’, 0.13626963),
(‘n02123394’, ‘Persian_cat’, 0.056125946)]]
tabbyはトラ猫だそうです。
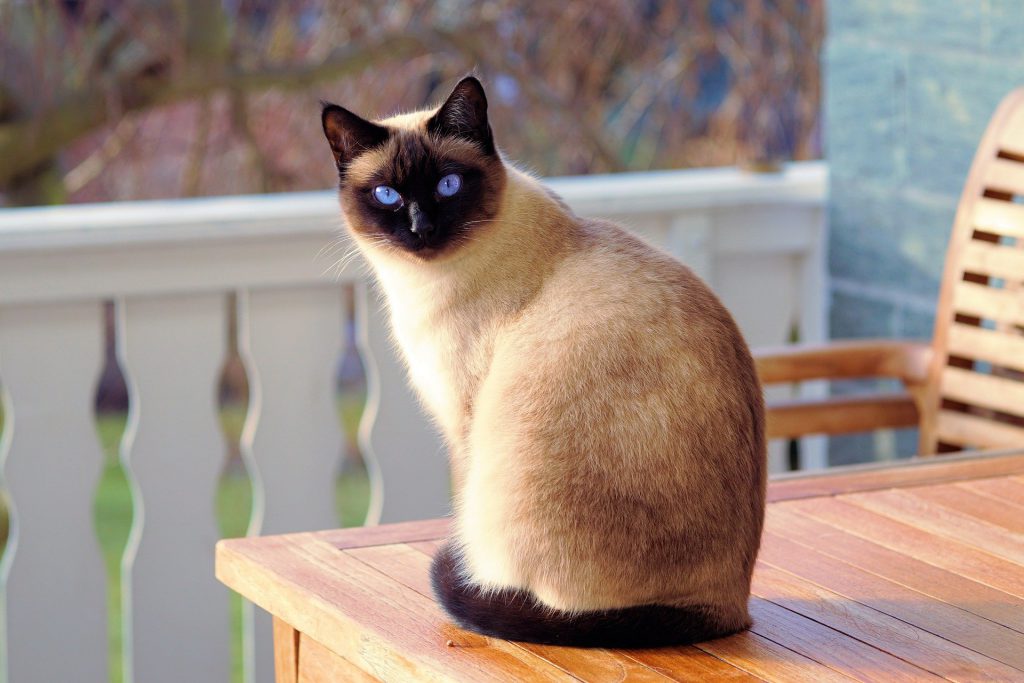
[[(‘n02123597’, ‘Siamese_cat’, 0.829578),
(‘n01877812’, ‘wallaby’, 0.012674228),
(‘n02497673’, ‘Madagascar_cat’, 0.008706838),
(‘n02500267’, ‘indri’, 0.007321883),
(‘n02127052’, ‘lynx’, 0.0048339292)]]
さてこれはどうでしょう。犬も猫も一緒です。
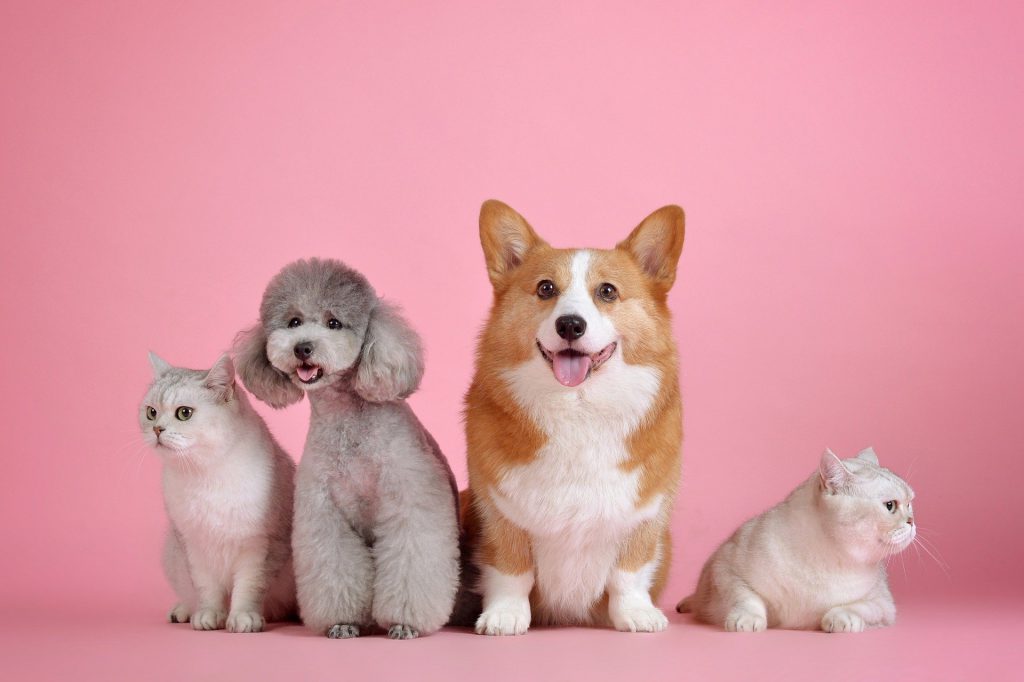
[[(‘n02113023’, ‘Pembroke’, 0.8427573),
(‘n02113186’, ‘Cardigan’, 0.027167425),
(‘n02094258’, ‘Norwich_terrier’, 0.007881726),
(‘n02110806’, ‘basenji’, 0.0076770447),
(‘n02110185’, ‘Siberian_husky’, 0.0065252013)]]
コーギーだけ当たってます。猫は??
1000カテゴリは何があるか
Efficient netの学習した1000カテゴリは以下で確認できます。
https://storage.googleapis.com/download.tensorflow.org/data/imagenet_class_index.json
犬 125種類
n02085620 | Chihuahua |
n02085782 | Japanese_spaniel |
n02085936 | Maltese_dog |
n02086079 | Pekinese |
n02086240 | Shih-Tzu |
n02086646 | Blenheim_spaniel |
n02086910 | papillon |
n02087046 | toy_terrier |
n02087394 | Rhodesian_ridgeback |
n02088094 | Afghan_hound |
n02088238 | basset |
n02088364 | beagle |
n02088466 | bloodhound |
n02088632 | bluetick |
n02089078 | black-and-tan_coonhound |
n02089867 | Walker_hound |
n02089973 | English_foxhound |
n02090379 | redbone |
n02090622 | borzoi |
n02090721 | Irish_wolfhound |
n02091032 | Italian_greyhound |
n02091134 | whippet |
n02091244 | Ibizan_hound |
n02091467 | Norwegian_elkhound |
n02091635 | otterhound |
n02091831 | Saluki |
n02092002 | Scottish_deerhound |
n02092339 | Weimaraner |
n02093256 | Staffordshire_bullterrier |
n02093428 | American_Staffordshire_terrier |
n02093647 | Bedlington_terrier |
n02093754 | Border_terrier |
n02093859 | Kerry_blue_terrier |
n02093991 | Irish_terrier |
n02094114 | Norfolk_terrier |
n02094258 | Norwich_terrier |
n02094433 | Yorkshire_terrier |
n02095314 | wire-haired_fox_terrier |
n02095570 | Lakeland_terrier |
n02095889 | Sealyham_terrier |
n02096051 | Airedale |
n02096177 | cairn |
n02096294 | Australian_terrier |
n02096437 | Dandie_Dinmont |
n02096585 | Boston_bull |
n02097047 | miniature_schnauzer |
n02097130 | giant_schnauzer |
n02097209 | standard_schnauzer |
n02097298 | Scotch_terrier |
n02097474 | Tibetan_terrier |
n02097658 | silky_terrier |
n02098105 | soft-coated_wheaten_terrier |
n02098286 | West_Highland_white_terrier |
n02098413 | Lhasa |
n02099267 | flat-coated_retriever |
n02099429 | curly-coated_retriever |
n02099601 | golden_retriever |
n02099712 | Labrador_retriever |
n02099849 | Chesapeake_Bay_retriever |
n02100236 | German_short-haired_pointer |
n02100583 | vizsla |
n02100735 | English_setter |
n02100877 | Irish_setter |
n02101006 | Gordon_setter |
n02101388 | Brittany_spaniel |
n02101556 | clumber |
n02102040 | English_springer |
n02102177 | Welsh_springer_spaniel |
n02102318 | cocker_spaniel |
n02102480 | Sussex_spaniel |
n02102973 | Irish_water_spaniel |
n02104029 | kuvasz |
n02104365 | schipperke |
n02105056 | groenendael |
n02105162 | malinois |
n02105251 | briard |
n02105412 | kelpie |
n02105505 | komondor |
n02105641 | Old_English_sheepdog |
n02105855 | Shetland_sheepdog |
n02106030 | collie |
n02106166 | Border_collie |
n02106382 | Bouvier_des_Flandres |
n02106550 | Rottweiler |
n02106662 | German_shepherd |
n02107142 | Doberman |
n02107312 | miniature_pinscher |
n02107574 | Greater_Swiss_Mountain_dog |
n02107683 | Bernese_mountain_dog |
n02107908 | Appenzeller |
n02108000 | EntleBucher |
n02108089 | boxer |
n02108422 | bull_mastiff |
n02108551 | Tibetan_mastiff |
n02108915 | French_bulldog |
n02109047 | Great_Dane |
n02109525 | Saint_Bernard |
n02109961 | Eskimo_dog |
n02110063 | malamute |
n02110185 | Siberian_husky |
n02110341 | dalmatian |
n02110627 | affenpinscher |
n02110806 | basenji |
n02110958 | pug |
n02111129 | Leonberg |
n02111277 | Newfoundland |
n02111500 | Great_Pyrenees |
n02111889 | Samoyed |
n02112018 | Pomeranian |
n02112137 | chow |
n02112350 | keeshond |
n02112706 | Brabancon_griffon |
n02113023 | Pembroke |
n02113186 | Cardigan |
n02113624 | toy_poodle |
n02113712 | miniature_poodle |
n02113799 | standard_poodle |
n02113978 | Mexican_hairless |
n02114367 | timber_wolf |
n02114548 | white_wolf |
n02114712 | red_wolf |
n02114855 | coyote |
n02115641 | dingo |
n02115913 | dhole |
n02116738 | African_hunting_dog |
猫 5種
n02123045 | tabby |
n02123159 | tiger_cat |
n02123394 | Persian_cat |
n02123597 | Siamese_cat |
n02124075 | Egyptian_cat |